- Published on
Python File Handling
- Authors
- Name
- Sanjeev Sharma
- @webcoderspeed1
Introduction
File handling is a crucial aspect of programming, allowing developers to interact with files on their computer systems. Whether it's reading data from a file, writing information to it, or modifying its contents, Python provides a robust set of tools for file handling.
- Working With File
- Opening and Closing File
- Common File Method
- Reading from a File
- Writing to a File
- More Examples
- Random Access in File - [File Positions]
- Conclusion
- FAQs
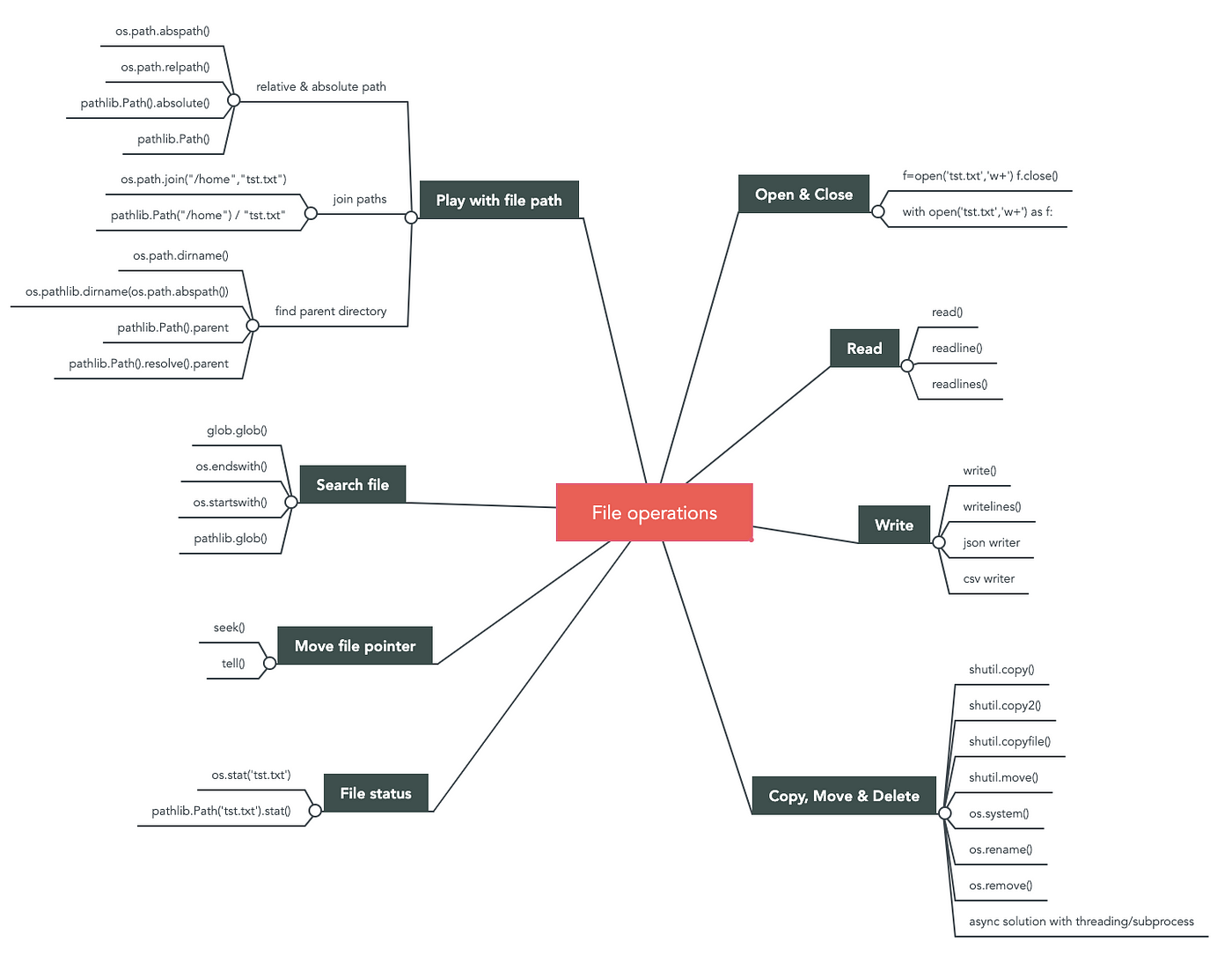
Working With File
Opening and Closing File
To work with a file in Python, the first step is to open it using the open() function. The open() function takes two arguments - the file name and the mode in which you want to open the file.
Modes include:
- 'r': Read (default mode)
- 'w': Write
- 'a': Append
- 'b': Binary mode
- 'x': Exclusive creation (fails if the file already exists)
- 't': Text mode (default mode)
- 'r+': Open for reading and writing start at beginning of file
- 'w+': Open for writing mode. Truncates the file (effectively overwritting it). If the file doesn't exist, it will attempt to create the file it delete all.
Common File Method
- write()
Syntax:
- read() Syntax:
fileObject.read([count])
- close() Syntax:
fileObject.close()
Let's start with a simple example of opening and closing a file:
# Open a file in read mode
file_path = 'example.txt'
file = open(file_path, 'r')
# Perform operations on the file (read, write, etc.)
# Close the file
file.close()
It's essential to close the file after performing operations to free up system resources. Alternatively, you can use the with statement to automatically close the file when done:
with open(file_path, 'r') as file:
# Perform operations on the file (read, write, etc.)
# File is automatically closed outside the 'with' block
Reading from a File
Reading from a file is a common operation in programming. Python offers various methods to read data from a file. One common approach is to use the read() method:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
The read() method reads the entire content of the file as a single string. If you want to read the file line by line, you can use the readline() or readlines() methods:
with open('example.txt', 'r') as file:
# Read a single line
line = file.readline()
print(line)
# Read all lines into a list
lines = file.readlines()
for line in lines:
print(line)
Writing to a File
To write to a file, open it in write or append mode ('w' or 'a'). The write() method is then used to add content to the file:
with open('output.txt', 'w') as file:
file.write('Hello, this is a sample text.\n')
file.write('Writing to a file in Python is easy!')
If you open the file in write mode ('w'), it will overwrite the existing content. If you want to add content without overwriting, use append mode ('a').
More Examples
############################################################
fp = open("file.txt", "w")
first = int(input("Enter First Number: "))
second = int(input("Enter Second Number: "))
s = f"div of {first} and {second}={first//second}\n"
if(fp.write(s)):
print("data written successfully!")
fp.close()
############################################################
fp = open("file.txt", "r")
print(fp.read(
fp.close()
############################################################
fp =open("file.txt", "r")
rpint(fp.read(10)) # count 10 no. of character
input()
print(fp.read())
fp.close()
############################################################
fp = open("file.txt", "r")
print(fp.readline())
fp.close()
############################################################
fp = open("file.txt", "r")
print(fp.readline())
fp.close()
############################################################
fp = open("file.txt", "r")
print(fp.read())
print(fp.read())
fp.close()
############################################################
fp = open("file.txt", "r")
print(fp.read())
fp.seck(0)
print(fp.read())
fp.close()
############################################################
fp = open("file.txt", "r")
print(fp.readlines())
fp.close()
############################################################
fp = open("file.txt", "r")
lst = fp.readlines()
for i in lst:
print(i, end=" ")
fp.close()
############################################################
fp = open("file.txt", "w")
lst = ["speed", "nik", "fury"]
fp.writelines(lst) # string only
fp.close()
############################################################
#### WRITING FROM ONE TO ANOTHER ####
fr = open("read.txt", "r")
fw = open("write.txt", "w")
for i in fr.readlines():
fw.write(i)
fr.close()
fw.close()
############################################################
fp = open("file.txt", "r+")
print(fp.read())
fp.write("This is a new line\n")
fp.seek(0)
print(fp.read())
fp.close()
############################################################
fp = open("file.txt", "r+")
fp.write("Blinkle")
fp.seek(0)
print(fp.read())
fp.close()
Random Access in File - [File Positions]
1 - Byte - one character
- tell()
- seek(offset[, from])
- from - 0 - Beginning position
- 1 - Current Position
- 2 - Ending Position
Note: We can only move back & forth in binary mode (rb)
Conclusion
In this journey through Python's file handling, we've covered the essentials and explored advanced techniques. Armed with this knowledge, developers can confidently tackle file manipulation tasks, unleashing Python's full potential.
FAQs
- Is file handling exclusive to Python?
No, file handling is a common concept in many programming languages, but Python's syntax makes it particularly user-friendly.
- What is the significance of the 'with' statement in file handling?
The 'with' statement ensures proper resource management, automatically closing files when the block of code is exited.
- How does Python handle errors in file operations?
Python uses try-except blocks to handle errors, allowing developers to gracefully manage unexpected issues.
- Can I manipulate files in different formats within the same program?
Yes, Python's versatility allows developers to handle various file formats seamlessly in a single program.
- Are there any security considerations when working with files in Python?
Yes, developers should validate user inputs and implement proper error handling to enhance the security of file handling operations.